Selenium is a powerful tool for automating web browsers, widely used for testing web applications and scraping data. When combined with Proxy302, a proxy service that supports session-based authentication, Selenium becomes even more versatile for tasks like bypassing IP restrictions or managing multiple sessions. This guide will explain what Selenium is and how to integrate Proxy302 with it effectively.
What is Selenium?
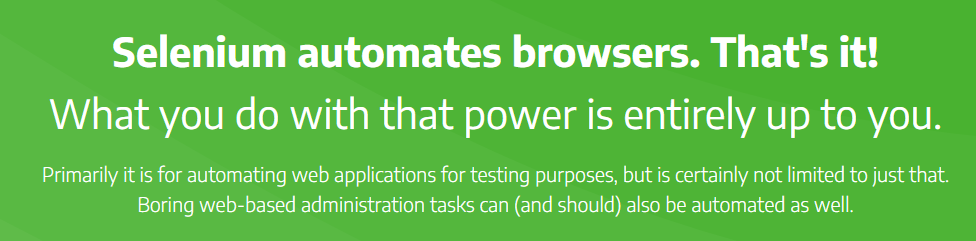
Selenium is an open-source framework that allows developers and testers to automate browser actions such as clicking buttons, filling forms, and navigating pages. It supports multiple programming languages (e.g., Python, Java, C#) and browsers (e.g., Chrome, Firefox, Edge). Key features include:
- Cross-Browser Testing: Test applications across different browsers and versions.
- Web Scraping: Extract data from websites efficiently.
- Automation: Automate repetitive tasks like form submissions or data entry.
What is Proxy302?
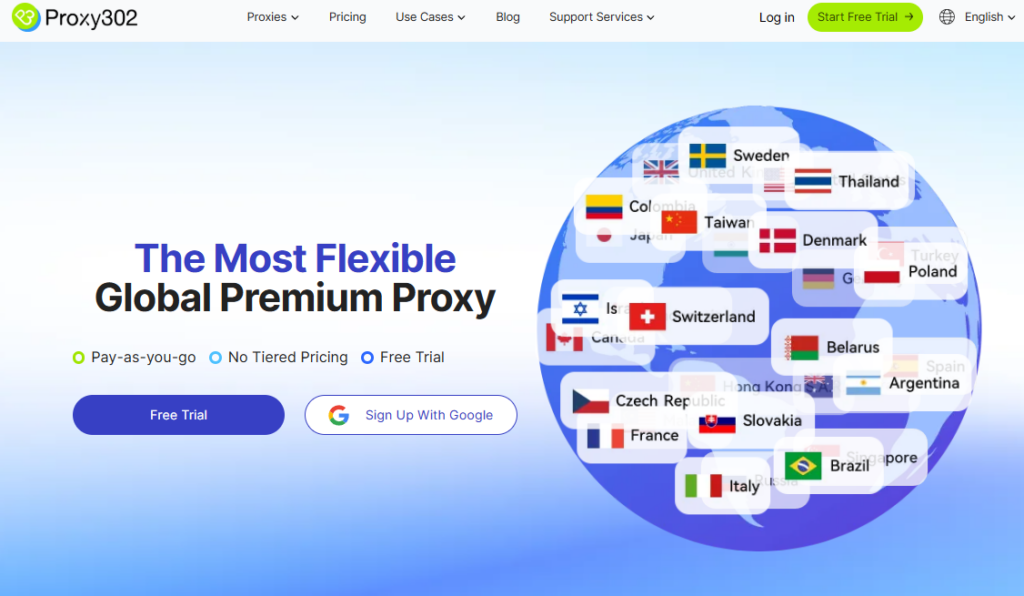
Proxy302 is a proxy service that supports session-based authentication, allowing users to manage multiple sessions or bypass IP restrictions. It is particularly useful for web scraping and testing scenarios where IP rotation or session management is required.
How to Use Proxy302 with Selenium
1. Install Selenium and Browser Drivers
- Install Selenium using pip:
pip install selenium
preview - Download the appropriate browser driver (e.g., ChromeDriver for Chrome) and ensure it matches your browser version.
2. Configure Proxy302 in Selenium
To integrate Proxy302 with Selenium, you need to pass the proxy details (IP, port, username, and password) in the browser options. Here’s an example in Python:
from selenium import webdriver
# Proxy302 details
proxy_ip = "your_proxy_ip"
proxy_port = "your_proxy_port"
proxy_username = "your_username"
proxy_password = "your_password"
# Configure proxy
proxy = f"{proxy_username}:{proxy_password}@{proxy_ip}:{proxy_port}"
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument(f"--proxy-server=http://{proxy}")
# Launch browser with proxy
driver = webdriver.Chrome(options=chrome_options)
driver.get("https://example.com")
3. Handle Session-Based Authentication
Proxy302 supports session-based authentication, which can be managed by passing session parameters in the request. For example:
# Add session parameters to the request
session_param = "your_session_id"
driver.get(f"https://example.com?session={session_param}")
4. Test and Debug
- Run your Selenium script and monitor its behavior.
- Use logging or debugging tools to troubleshoot any issues with proxy integration.
Use Cases of Selenium with Proxy302
- Web Scraping: Bypass IP restrictions and scrape data from websites without getting blocked.
- Testing: Simulate multiple user sessions or IP addresses for comprehensive testing.
- Automation: Automate tasks that require session management or IP rotation.
Best Practices
- Rotate Proxies: Use multiple Proxy302 IPs to avoid detection and bans.
- Handle Errors Gracefully: Implement error handling for connection issues or authentication failures.
- Optimize Performance: Use headless mode or lightweight browsers for faster execution.
Conclusion
Selenium, combined with Proxy302, is a powerful solution for web scraping, testing, and automation. By following the steps above, you can seamlessly integrate Proxy302 into your Selenium workflows and unlock advanced capabilities like session management and IP rotation.
Ready to get started? Install Selenium today and explore the possibilities with Proxy302!
👉 Start Your Free Trial Now 👈and unlock a world without digital borders.
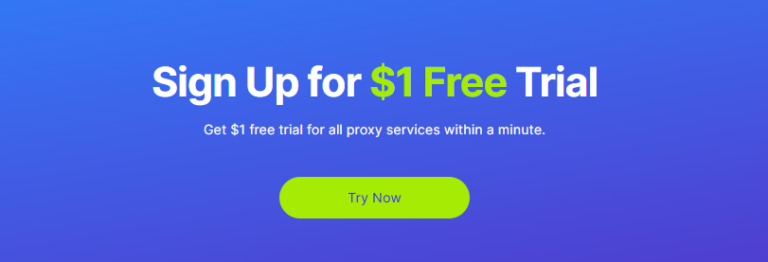
3 comments
The way Proxy302 enhances Selenium for session management and bypassing IP restrictions is a real game-changer for web scraping. It’s exciting to see how these tools can work together to tackle those anti-bot measures effectively.
The explanation of Selenium’s capabilities alongside Proxy302’s session handling is helpful. A quick tip for readers: always handle proxy timeouts and retries gracefully in your script to avoid unexpected failures.
I’ve been using Selenium for testing, but hadn’t considered combining it with session-based proxies. This seems like a solid solution for managing concurrent scraping threads without hitting rate limits.