In the world of web development and data gathering, using a proxy can be as essential as having a good internet connection. Proxies serve as intermediaries between your computer and the internet, allowing you to browse anonymously, access geo-restricted content, and manage multiple requests efficiently. If you’re using Python, the requests
library is a popular choice for making HTTP requests. But how do you integrate proxies with this library? This guide will walk you through the process step-by-step, with a focus on using Proxy302, a service that offers over 65 million IPs globally, comprehensive proxy types including city-level targeting residential proxies, and a flexible pay-as-you-go model.
What is a Proxy?
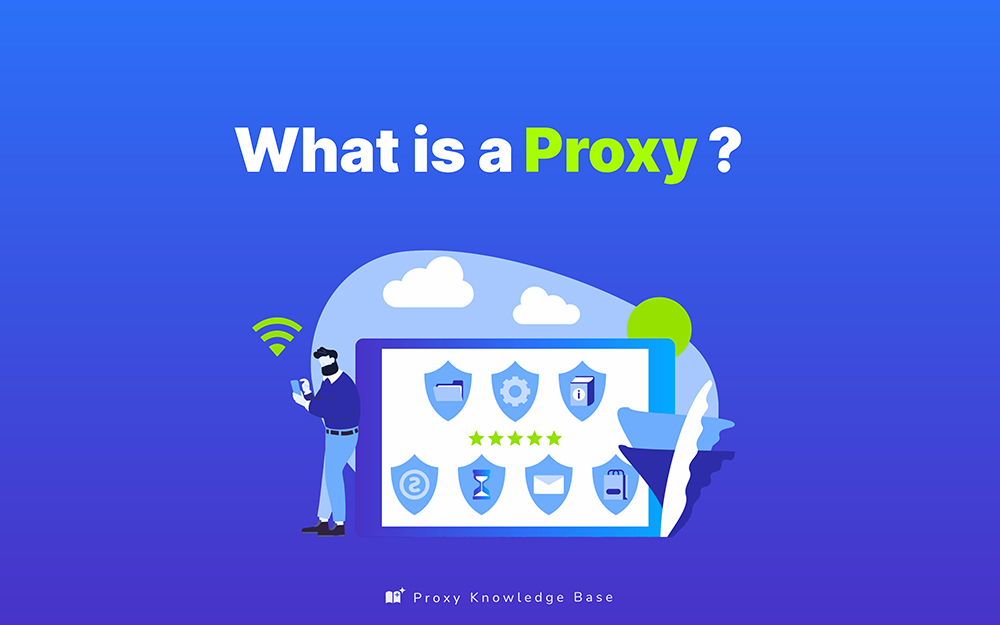
A proxy server acts as a gateway between you and the internet. It’s like having a middleman handle your requests to the web, which can be beneficial for privacy, security, and access control. Proxies come in various forms, including:
- HTTP Proxies: Ideal for web traffic.
- HTTPS Proxies: Secure version of HTTP proxies, encrypting data for privacy.
- SOCKS Proxies: More versatile, can handle any kind of traffic, but generally slower.
Why Use Proxies with Python Requests?
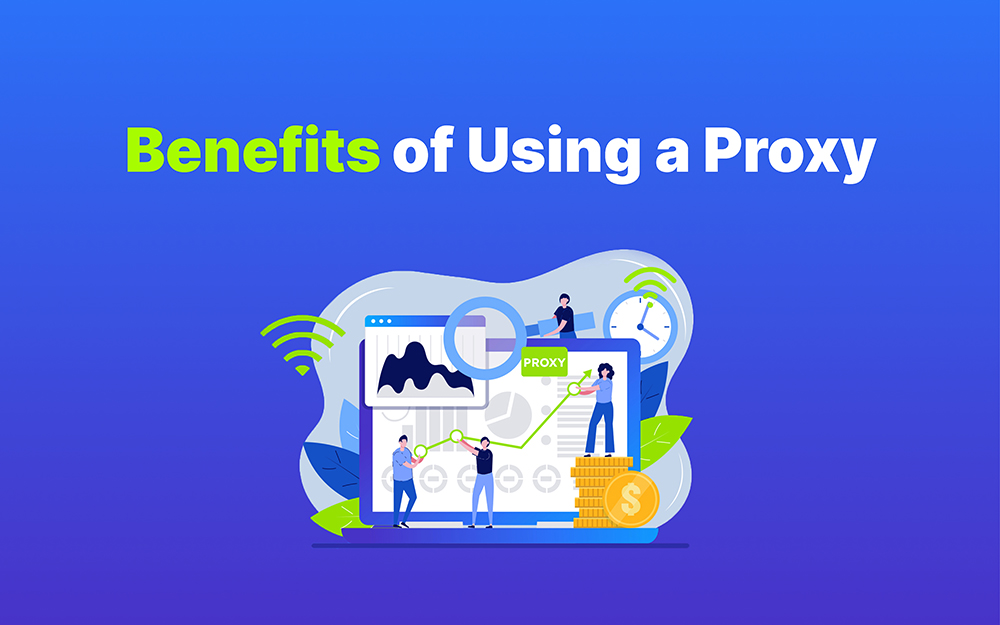
Imagine trying to access a website that restricts content based on location. Without a proxy, you’re stuck. Proxies can help you bypass these restrictions by masking your IP address. Here are a few reasons to use proxies with Python requests:
- Anonymity: Protect your identity by hiding your IP address.
- Access Control: Bypass geo-restrictions and access content available in different regions.
- Load Balancing: Distribute requests across multiple IPs to prevent server overload.
- Web Scraping: Avoid getting blocked by websites when scraping data by rotating IPs.
Setting Up Your Environment
Before diving into code, ensure your environment is set up correctly.
Installing the Requests Library
First, make sure you have Python installed. You can download it from the official website. Then, install the requests
library using pip:
pip install requests
This command will download and install the latest version of the requests
library, which is essential for making HTTP requests in Python.
Configuring Proxies in Python Requests
Now, let’s get hands-on with configuring a proxy using the requests
library.
Basic Proxy Setup
Setting up a proxy is straightforward. You simply need to define a dictionary with your proxy settings and pass it to the requests
methods.
import requests
proxy = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
url = 'http://example.com'
response = requests.get(url, proxies=proxy)
print(response.text)
In this example, replace 'http://10.10.1.10:3128'
with your actual proxy address. This setup will route your requests through the specified proxy.
Leveraging Proxy302 Features
With Proxy302, you gain access to over 65 million IPs globally. This vast network allows for city-level targeting, which is crucial for localized data collection. Their pay-as-you-go model eliminates the need for monthly subscriptions, providing flexibility and cost efficiency.
Handling Proxy Authentication
Some proxies require authentication. Here’s how to handle it:
proxy = {
'http': 'http://username:[email protected]:3128',
'https': 'http://username:[email protected]:1080',
}
Replace 'username:password'
with your credentials. Proxy302 supports authenticated proxies, ensuring secure and reliable connections.
Using Environment Variables for Proxy Configuration
For a more global approach, you can set your proxy via environment variables. This method applies the proxy settings to all requests made by the requests
library.
import os
os.environ['HTTP_PROXY'] = 'http://10.10.1.10:3128'
os.environ['HTTPS_PROXY'] = 'http://10.10.1.10:1080'
This setup is particularly useful for applications that require consistent proxy usage across multiple modules.
Configuring Proxies in Python Requests
Now, let’s get hands-on with configuring a proxy using the requests
library.
Basic Proxy Setup
Setting up a proxy is straightforward. You simply need to define a dictionary with your proxy settings and pass it to the requests
methods.
import requests
proxy = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
url = 'http://example.com'
response = requests.get(url, proxies=proxy)
print(response.text)
In this example, replace 'http://10.10.1.10:3128'
with your actual proxy address. This setup will route your requests through the specified proxy.
Leveraging Proxy302 Features
With Proxy302, you gain access to over 65 million IPs globally. This vast network allows for city-level targeting, which is crucial for localized data collection. Their pay-as-you-go model eliminates the need for monthly subscriptions, providing flexibility and cost efficiency.
Handling Proxy Authentication
Some proxies require authentication. Here’s how to handle it:
proxy = {
'http': 'http://username:[email protected]:3128',
'https': 'http://username:[email protected]:1080',
}
Replace 'username:password'
with your credentials. Proxy302 supports authenticated proxies, ensuring secure and reliable connections.
Using Environment Variables for Proxy Configuration
For a more global approach, you can set your proxy via environment variables. This method applies the proxy settings to all requests made by the requests
library.
import os
os.environ['HTTP_PROXY'] = 'http://10.10.1.10:3128'
os.environ['HTTPS_PROXY'] = 'http://10.10.1.10:1080'
This setup is particularly useful for applications that require consistent proxy usage across multiple modules.
Error Handling and Best Practices
Error Handling
When working with proxies, you might encounter errors such as timeouts or connection refusals. Here’s a simple error handling example:
try:
response = requests.get(url, proxies=proxy)
response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
This code will catch any exceptions raised during the request and print an error message, helping you diagnose issues quickly.
Best Practices
- Rotate Proxies: Regularly change your proxy to avoid detection and blocking.
- Test Proxies: Before deploying, test your proxies to ensure they’re working correctly.
- Respect Terms of Service: Always comply with the terms of service of the websites you’re accessing.
Conclusion
Using proxies with Python requests is a powerful way to enhance your web interactions, whether for web scraping, accessing geo-restricted content, or maintaining anonymity. With Proxy302, you have access to a vast network of IPs, comprehensive proxy types, and a flexible payment model, making it an excellent choice for both beginners and advanced users.
Additional Resources
Ready to take your proxy usage to the next level? 👉 Start Your Free Trial Now 👈to get you started. With no tiered pricing and a pay-as-you-go model, you can explore the benefits without commitment. Visit Proxy302 to learn more and start your free trial today!
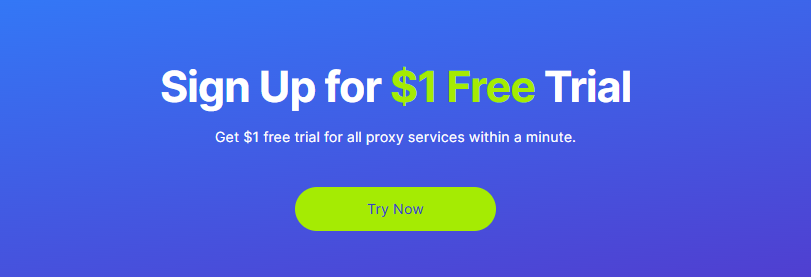
8 comments
With havin so much written content do you ever run into any issues of plagorism or copyright infringement?
My blog has a lot of exclusive content I’ve either created myself or outsourced but it looks like a lot of it is popping it up
all over the web without my authorization. Do you know any methods to help stop content from being stolen? I’d definitely appreciate it.
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Thank you for taking the time to write this! It’s always great to find high-quality content like this.
I’m curious to find out what blog platform you
happen to be working with? I’m having some minor security issues
with my latest blog and I’d like to find something more risk-free.
Do you have any recommendations?
หลังจากที่ผู้ใช้ทำการลงทะเบียนแล้ว สามารถเข้าสู่ระบบได้โดยการใช้ชื่อผู้ใช้และรหัสผ่านที่ได้ตั้งไว้ในขั้นตอนการสมัคร เมื่อเข้าสู่ระบบเรียบร้อยแล้ว ผู้เล่นสามารถเข้าถึงเกมคาสิโนออนไลน์ที่หลากหลาย ไม่ว่าจะเป็นสล็อต คาสิโนสด หรือกีฬา และยังสามารถใช้โปรโมชั่นและโบนัสที่มีให้แก่ผู้ใช้ใหม่ได้ทันที
Hi there, i read your blog from time to time and i own a similar one and i was just wondering if you get a lot of spam comments? If so how do you stop it, any plugin or anything you can advise? I get so much lately it’s driving me crazy so any support is very much appreciated.